Data Preprocessing in Machine Learning:
In the world of machine learning, data preprocessing plays a critical role in shaping the foundation of a successful model. It involves transforming raw data into a suitable format for machine learning algorithms, ensuring that the data is consistent, clean, and ready for analysis. In this article, we will dive deep into the various steps of data preprocessing, complete with code examples, to help you understand the process thoroughly.
1. Getting the Dataset: The first step in any machine learning project is obtaining the dataset that you will be working with. The dataset could be sourced from various places such as public repositories, databases, APIs, or even manually collected data. Once you have your dataset, you can begin the journey of preparing it for analysis.
2. Importing Libraries: Python’s ecosystem boasts a rich selection of libraries for data preprocessing. For this guide, we’ll make use of three fundamental ones: pandas for data manipulation, numpy for numerical operations, and scikit-learn for machine learning functions.
Python Code
import numpy as np
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import LabelEncoder, StandardScaler
3. Importing Datasets: Let’s assume you have your dataset stored in a CSV file named ‘dataset.csv’. To load this data into memory, pandas comes to the rescue.
Python Code
data = pd.read_csv(‘dataset.csv’)
# Display the first few rows of the dataset
print(data.head()
4. Finding Missing Data: Missing data is an inevitable hurdle when dealing with real-world datasets. Detecting and addressing missing values is crucial to prevent skewed analysis and inaccurate models. Let’s identify missing values and replace them with the mean of the respective column.
Python Code
missing_values = data.isnull().sum()
print(“Missing Values:\n”, missing_values)
# Impute missing values (replace with mean)
data.fillna(data.mean(), inplace=True)
# Display the first few rows of the modified dataset
print(data.head())
5. Encoding Categorical Data: Most machine learning algorithms operate on numerical data, but real-world datasets often contain categorical variables like gender, city, or product type. These variables must be transformed into numerical form.
- Label Encoding: For categorical variables with only two unique values, label encoding is a suitable choice.
Python Code
label_encoder = LabelEncoder()
data[‘Gender’] = label_encoder.fit_transform(data[‘Gender’])
# Display the first few rows of the modified dataset
print(data.head())
- One-Hot Encoding: For categorical variables with more than two categories, one-hot encoding is recommended. This creates binary columns for each category.
Python Code
data = pd.get_dummies(data, columns=[‘City’])
# Display the first few rows of the modified dataset
print(data.head())
6. Splitting Dataset into Training and Test Set: To assess your machine learning model’s performance, you need to test it on unseen data. Splitting your dataset into training and test sets accomplishes this.
Python Code
X = data.drop(‘Purchased’, axis=1)
y = data[‘Purchased’]
# Split into training and test sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Display the shape of training and test sets
print(“X_train shape:”, X_train.shape)
print(“X_test shape:”, X_test.shape)
7. Feature Scaling: Machine learning algorithms often perform better when features are on the same scale. Two common scaling techniques are Min-Max scaling and Standardization.
- Standardization: Standardization transforms features to have zero mean and unit variance.
Python Code
scaler = StandardScaler()
X_train_scaled = scaler.fit_transform(X_train)
X_test_scaled = scaler.transform(X_test)
# Display the scaled features
print(“Scaled Training Features:\n”, X_train_scaled)
print(“Scaled Test Features:\n”, X_test_scaled)
In conclusion, data preprocessing is the cornerstone of successful machine learning projects. By following these steps—getting the dataset, importing libraries, importing datasets, handling missing data, encoding categorical data, splitting the dataset, and performing feature scaling—you lay a solid foundation for building powerful and accurate machine learning models. Armed with this knowledge and the provided code examples, you’re ready to tackle a wide range of real-world data preprocessing challenges. Remember, a well-preprocessed dataset is the bedrock of predictive modeling success.
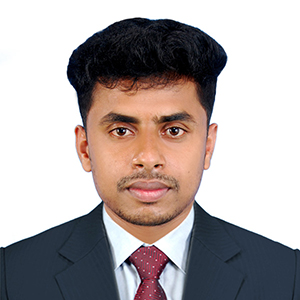
Assistant Teacher at Zinzira Pir Mohammad Pilot School and College