Explain the Main Parts of a Process:
A process is an independent program in execution, comprising the program code, associated data, and the execution context. It is the basic unit of execution in a computer system. Each process runs in its own address space, isolated from other processes, and has its own resources, such as registers, program counter, and file handles. Processes provide a way for the operating system to manage and execute multiple tasks concurrently. Here we explain the main parts of a process:
1. Program Counter (PC):
Function: The Program Counter is a special register within the Central Processing Unit (CPU) that keeps track of the memory address of the next instruction to be executed in the program.
Role: Its primary role is to maintain the sequence of instructions, ensuring that the CPU fetches the correct instruction from memory for execution.
Importance: Without a functioning Program Counter, the CPU would lack a reference point for instruction execution, leading to chaotic and unpredictable behavior in program execution.
2. Registers:
Function: Registers are small, high-speed storage locations embedded within the CPU. They hold data temporarily during the execution of machine instructions.
Role: By providing quick access to data, registers significantly enhance the speed of computations. They store intermediate results, operands, and memory addresses, facilitating efficient data manipulation by the CPU.
Types: General-purpose registers (e.g., for arithmetic operations) and special-purpose registers (e.g., for the program counter or specific CPU control functions) contribute to the versatility and functionality of the CPU.
3. Stack:
Function: The stack is a region of memory set aside for dynamic storage during the execution of a program. It operates on the Last-In-First-Out (LIFO) principle, meaning the last item placed on the stack is the first one to be removed.
Role: The stack is crucial for managing function calls and local variables. When a function is called, its local variables and return address are pushed onto the stack, and they are popped off when the function returns, maintaining the correct execution context.
Usage: The stack grows and shrinks as functions are called and return, providing a systematic way to handle function invocations and returns.
4. Heap:
Function: The heap is a dynamic memory allocation area separate from the stack, used for storing data structures whose size is not known at compile time.
Role: Unlike the stack, which operates in a predictable and managed manner, the heap allows for flexible memory allocation and deallocation during runtime. This is especially important for data structures like arrays and linked lists whose size can change dynamically.
Usage: Memory on the heap must be explicitly managed by the programmer, and failure to do so can lead to memory leaks or inefficient memory usage.
5. Data Section:
Function: The Data Section of a process’s memory contains global and static variables that persist throughout the program’s execution.
Role: It serves as a centralized location for storing variables that need to retain their values across different function calls. Global variables are accessible by any function in the program.
Usage: The Data Section provides a way to share data across different parts of the program and maintains the state of variables throughout the program’s lifespan.
6. Code Section:
Function: The Code Section, also known as the Text Section, holds the executable instructions of the program in machine code.
Role: It contains the actual program logic and algorithms, providing the instructions that the CPU fetches, decodes, and executes.
Usage: The Code Section is typically read-only during program execution and is loaded into memory when the program starts. The Program Counter points to instructions within this section.
7. Process Control Block (PCB):
Function: The Process Control Block (PCB) is a data structure maintained by the operating system for each process. It stores essential information about the state of a process.
Role: The PCB includes details such as register values, program counter, process ID, priority, and other relevant information. It enables the operating system to manage and control processes effectively.
Usage: The PCB is crucial for process scheduling, context switching, and resource allocation. It allows the operating system to save and restore the state of a process during its execution.
8. Instruction Register (IR):
Function: The Instruction Register (IR) is a special-purpose register that holds the current instruction being executed by the CPU.
Role: It facilitates the decoding and execution of machine code instructions. The CPU uses the information stored in the IR to carry out the specific operation indicated by the instruction.
Importance: The IR is essential for the proper execution of instructions, ensuring that the CPU performs the correct operations based on the fetched instruction.
9. Memory Management Unit (MMU):
Function: The Memory Management Unit (MMU) is responsible for translating virtual addresses used by a program into physical addresses in the computer’s memory.
Role: It enables processes to have their own virtual address space while efficiently using the available physical memory. The MMU plays a crucial role in memory protection and the implementation of virtual memory systems.
Usage: The MMU translates addresses during each memory access, ensuring proper isolation between processes and allowing the operating system to manage memory effectively.
10. I/O Buffers and Status Registers:
Function: I/O Buffers temporarily store data during input or output operations, while Status Registers provide information about the status of I/O devices.
Role: I/O Buffers help manage the flow of data between the CPU and external devices, preventing bottlenecks. Status Registers convey the operational status of devices, allowing the CPU to respond appropriately.
Usage: Efficient communication with peripherals is facilitated by I/O Buffers, and Status Registers aid in error handling and device control, contributing to overall system reliability.
These components collectively contribute to the proper functioning of a process within a computer system, ensuring the execution of programs and effective management of resources.
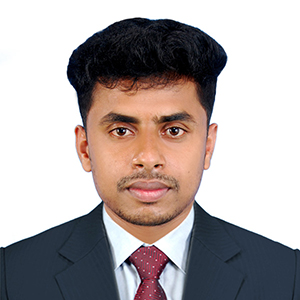
Former Student at Rajshahi University